Embedded Payment
Introduction
The Embedded Payment allows you to integrate a seamless payment experience directly on your website, enabling customers to choose from Card payments, Apple Pay, or Google Pay without leaving your site. With a single integration, you can offer a unified checkout interface that blends perfectly with your website’s design, providing a consistent and user-friendly experience. This ensures that customers can easily select their preferred payment method and complete transactions in just a few clicks.
In addition to the flexible payment options, the Embedded Payment takes care of all PCI DSS compliance requirements, so you don't need to worry about certification or security concerns. MyFatoorah handles the heavy lifting, allowing you to focus on delivering a smooth and secure payment process while maintaining full control over the checkout experience.
MyFatoorah's Embedded Payment is a JavaScript library that offers a single integration point for handling payments. With this library, customers can enter their card details directly into a form, and MyFatoorah takes care of processing the payment. Additionally, it supports Apple Pay and Google Pay with just a click, allowing MyFatoorah to smoothly handle the entire payment flow.
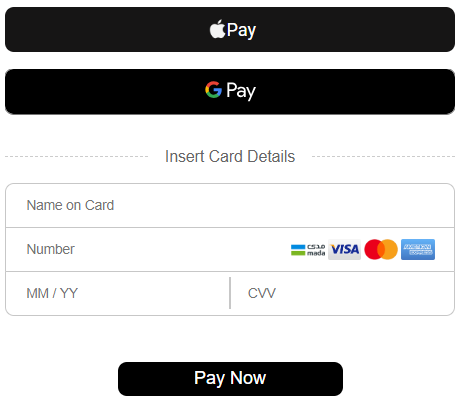
Default view of Embedded Payment
How it Works
1. Call InitiateSession endpoint
After you call the InitiateSession endpoint, you will get a SessionId and the CountryCode.
{
"IsSuccess": true,
"Message": "Initiated Successfully!",
"ValidationErrors": null,
"Data": {
"SessionId": "dfc6a3c3-df09-44cb-9c6a-0a6375752da6",
"CountryCode": "KWT",
"CustomerTokens": []
}
}
2. Include the Javascript library
Choose the test or the live library according to your working environment.
// Test Environment
<script src="https://demo.myfatoorah.com/payment/v1/session.js"></script>
// Live Environment Except Saudi Arabia and Qatar
<script src="https://portal.myfatoorah.com/payment/v1/session.js"></script>
// Live Environment For Saudi Arabia
<script src="https://sa.myfatoorah.com/payment/v1/session.js"></script>
// Live Environment For Qatar
<script src="https://qa.myfatoorah.com/payment/v1/session.js"></script>
// Live Environment For Egypt
<script src="https://eg.myfatoorah.com/payment/v1/session.js"></script>
3. Define a div element
You need to define a div element with a unique id attribute. The Unified Session will be loaded inside this div after passing the div id to the configuration variable.
<div id="embedded-payment"></div>
div elements
If you want to display each method (Card, GooglePay, ApplePay) in a separate div, you can create a div element for each method and display the methods in their corresponding div elements by adding it in the configuration of the payment method in Embedded Payment.
4. Configure the Embedded Payment
Now you need to add the configuration variables. Place the following snippet in a new script tag after loading the library in step 1, and replace the sessionId parameter with the "SessionId" you receive from the InitiateSession Endpoint.
For the countyCode parameter check our list of ISO Lookups.
var config = {
sessionId: "", //Add the "SessionId" you received from InitiateSession Endpoint.
countryCode: "", //Add the "CountryCode" you received from InitiateSession Endpoint.
currencyCode: "", //Here, you add the value of "PaymentCurrencyIso" for the gateways enabled that you received from InitiatePayment endpoint
amount: "", //This amount will be displayed on GooglePay and ApplePay
callback: payment,//MyFatoorah calls the callback function after the customer fills in the card and clicks pay or when the customer finishes the steps with GooglePay & ApplePay
containerId: "embedded-payment",//Enter the div id you created in Step 2
paymentOptions: ["ApplePay", "GooglePay", "Card"],//Enter the payment methods you want to display, Default value is "Card"
};
myFatoorah.init(config);
Apple Pay Prerequisites
To enable Apple Pay, you must verify your domain. For detailed instructions, please refer to our Apple Pay Domain Verification Guide.
5. Handle the callback function
After the customer completes the payment details entry, MyFatoorah will trigger the CallBack function. MyFatoorah returns to you the SessionId and details about the card used. You should send the SessionId to your backend to call ExecutePayment to complete the payment
function payment(response) {
//Pass session id to your backend here
if (response.isSuccess) {
switch (response.paymentType) {
case "ApplePay":
console.log("response >> " + JSON.stringify(response));
break;
case "GooglePay":
console.log("response >> " + JSON.stringify(response));
break;
case "Card":
console.log("response >> " + JSON.stringify(response));
break;
default:
console.log("Unknown payment type");
break;
}
}
};
6. Call the ExecutePayment Endpoint
Then, you need to send the SessionId to your server to process the actual transaction, which should be done in your backend environment using ExecutePayment endpoint.
Parameters conflict
Do not pass the PaymentMethodId parameter in the ExecutePayment request and use the SessionId parameter instead. As PaymentMethodId overwrite SessionId.
The "SessionId" you receive from InitiateSession Endpoint can not be used directly in ExecutePayment Endpoint.
You will get the PaymentURL in the Response Model. This payment URL is the 3D Secure page URL.
For Card and Google Pay, you can either redirect the customer to the PaymentUrl or open it in an iframe.
For Apple Pay, you can either invoke the PaymentUrl in the backend and get the status of the transaction either by the webhook or GetPaymentStatus, or you can open the PaymentUrl and the customer will be redirected to the CallBackUrl/ErrorUrl sent in the request to ExecutePayment.
{
"SessionId":"36c1bab2-1e21-ec11-bae9-000d3aaca798",
"InvoiceValue":10,
}
{
"IsSuccess":true,
"Message":"Invoice Created Successfully!",
"ValidationErrors":null,
"Data":{
"InvoiceId":1040089,
"IsDirectPayment":false,
"PaymentURL":"https://demo.MyFatoorah.com/En/KWT/PayInvoice/MpgsAuthentication?paymentId=0706104008982520266&sessionId=SESSION0002087297105E3203998J76",
"CustomerReference":"",
"UserDefinedField":null,
"RecurringId":""
}
}
Payment Status
To update your system automatically instead of manually following up with your customers via your portal account, you can use the Webhook feature or/and set call GetPaymentStatus after the customer is redirected to the CallBackUrl/ErrorUrl page. We recommend using the webhook and GetPaymentStatus together as a best practice.
Open the OTP page in an iframe
This feature enables you to open the OTP page in an iframe without external redirection. This will enable you to keep your customers on your website during the whole payment process.
How it Works
1. Create the iframe on your website.
The design and creation of the iframe are done from your side. You will open the PaymentUrl in the iframe so that the customer can enter their OTP.
Handling the iframe
You will handle fully the iframe from your side. You need to create the iframe and open the PaymentUrl for the OTP in it. You should also handle the cancelation if the customer wants to cancel the payment after the OTP is open and so on.
2. Get the redirection URL after the customer enters his OTP
After the customer completes the 3DS challenge, you will need to use an event listener to get the redirection URL. The event listener needs to be added to the page (In a javascript section) on which you will open the iframe.
The name of the sender of the message will be exactly "MF-3DSecure"
The redirection URL will be the CallBackUrl /ErrorUrl that you sent to ExecutePayment in the request appended to it the PaymentId.
https://{{Your_CallBackURL}}/?paymentId={{PaymentId}}&Id={{ID}}
You will have multiple options to choose from for the next action, whether you want to open the redirection URL in the iframe or close the iFrame and show the result page directly on your website.
//The event listener is used to listen to the Redirection URL after the customer completes the 3DS Challenge
window.addEventListener("message", function (event) {
if (!event.data) return;
try {
//The redirection URL is returned in the message
var message = JSON.parse(event.data);
//Proceed only with the following steps if the sender is exactly "MF-3DSecure"
if (message.sender == "MF-3DSecure") {
var url = message.url;
//Here, you need to handle the next action, and here are some suggestions:
//Redirect the full page to the received URL.
//Load the received URL in your iframe.
//Close the iframe and display the result on the same page. You can use AJAX requests to your server with the payment ID to confirm the transaction status (invoke GetPaymentStatus) and display the result accordingly.
}
} catch (error) {
return;
}
}, false);
Old embedded integration
If you have integrated with MyFatoorah using the old implementation where each payment method requires a different integration, this flow will still be available. Please find the documentation here:
Updated 16 days ago